Recently, I've been working on a large project in Rust, but my laptop isn't powerful enough to handle it efficiently. So, I decided to buy a new mini-PC specifically for this task.
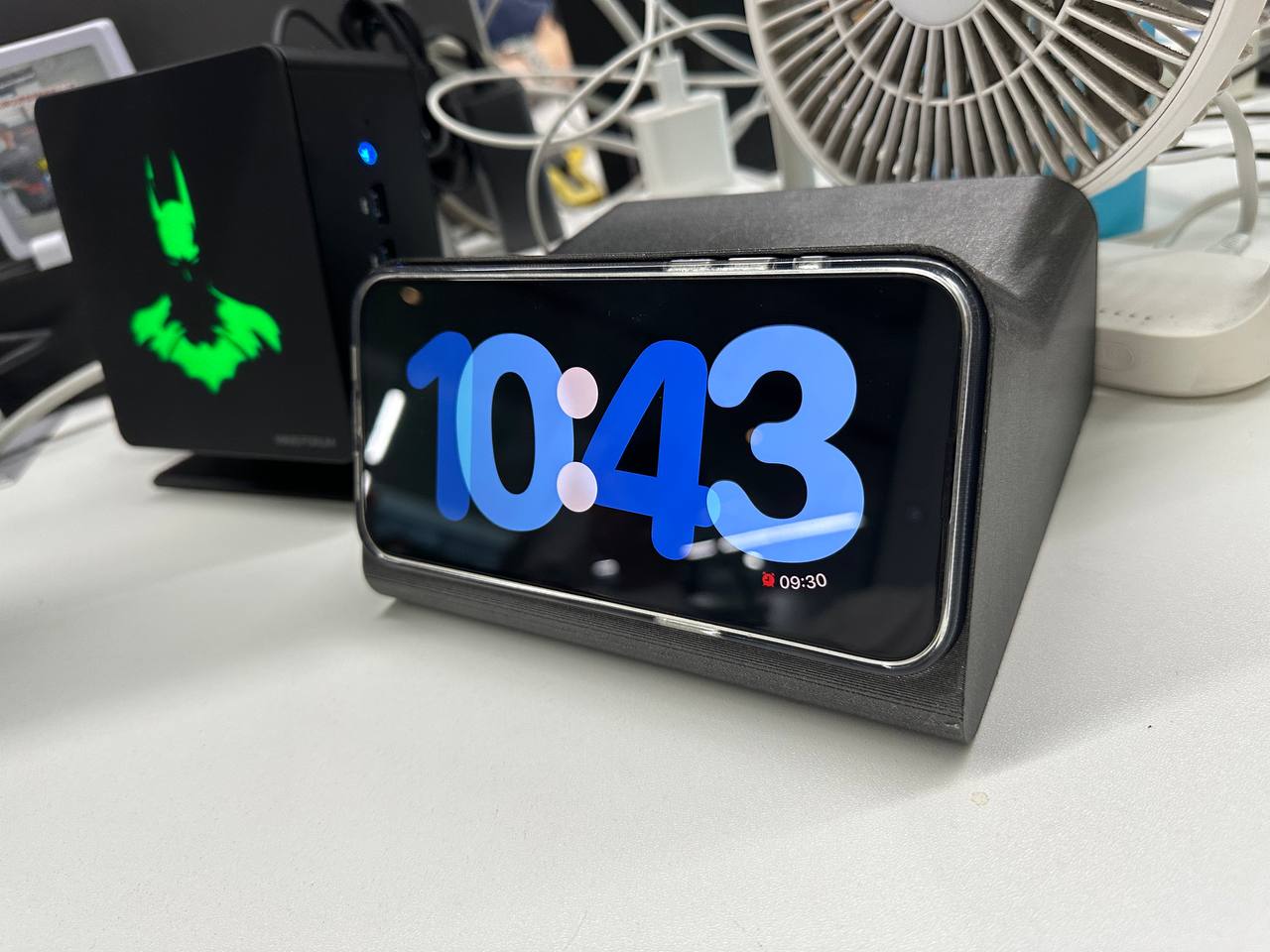
Since I’m still more accustomed to developing on macOS and didn’t want to go through the hassle of setting up a Hackintosh, I decided to install NixOS on my new mini PC. Initially, I planned to continue using VSCode for remote development, but later realized that Neovim is also a great option.